- Joined
- Jan 24, 2009
- Messages
- 786
- Reaction score
- 485
Few days ago our fellow vegan friend (@Dea7h) shared a web warehouse with the ability to drag & drop items in order to move them across 2 warehouses (e.g. Web warehouse -> In-game warehouse).
I've re-created his idea and made it generic, so it can be used in any web - the only thing that you will need is to implement the actual API call in the back-end to move the item
Demo
Usage
Download
Github Releases (use the latest version)
Source
I've re-created his idea and made it generic, so it can be used in any web - the only thing that you will need is to implement the actual API call in the back-end to move the item
Demo
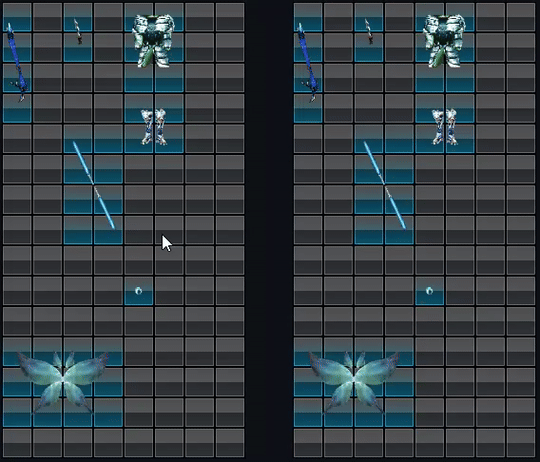
Usage
HTML:
<div class="storage-wrapper">
<div id="game-wh" class="item-storage game"></div>
<div id="web-wh" class="item-storage web"></div>
</div>
<script src="./mu-item-dragging.js"></script>
<script type="module">
// The items should be loaded from the database and should have img, pos {x, y} and size {x, y} properties.
// Array of items to be placed in the in-game warehouse
const gameItems = [
{ img: "/assets/items/1x4.png", pos: { x: 1, y: 1 }, size: { x: 1, y: 4 } },
{ img: "/assets/items/2x3.png", pos: { x: 5, y: 1 }, size: { x: 2, y: 3 } },
{ img: "/assets/items/2x4.png", pos: { x: 3, y: 5 }, size: { x: 2, y: 4 } },
{ img: "/assets/items/4x3.png", pos: { x: 1, y: 12 }, size: { x: 4, y: 3 } },
];
// Array of items to be placed in the web warehouse
const webItems = [
{ img: "/assets/items/1x1.png", pos: { x: 5, y: 10 }, size: { x: 1, y: 1 } },
{ img: "/assets/items/1x2.png", pos: { x: 3, y: 1 }, size: { x: 1, y: 2 } },
{ img: "/assets/items/2x2.png", pos: { x: 5, y: 4 }, size: { x: 2, y: 2 } },
];
// Create Storage Supervisor instance that will manage a group of warehouses
const supervisor = new StorageSupervisor({
moveItem(item, originStorage, targetStorage, slot) {
const isSameStorage = originStorage.compareWith(targetStorage);
// TODO: Make an API call to your web's back end to move the item.
// Reject the promise to prevent moving the item in the UI.
return Promise.resolve({
item,
slot,
from: originStorage.id,
to: targetStorage.id,
isOriginatingStorage: isSameStorage,
});
},
});
// Add a warehouse to the supervisor
await supervisor.addStorage("game-storage", { selector: "#game-wh", items: gameItems });
await supervisor.addStorage("web-storage", { selector: "#web-wh", items: webItems });
</script>
Download
Github Releases (use the latest version)
Source